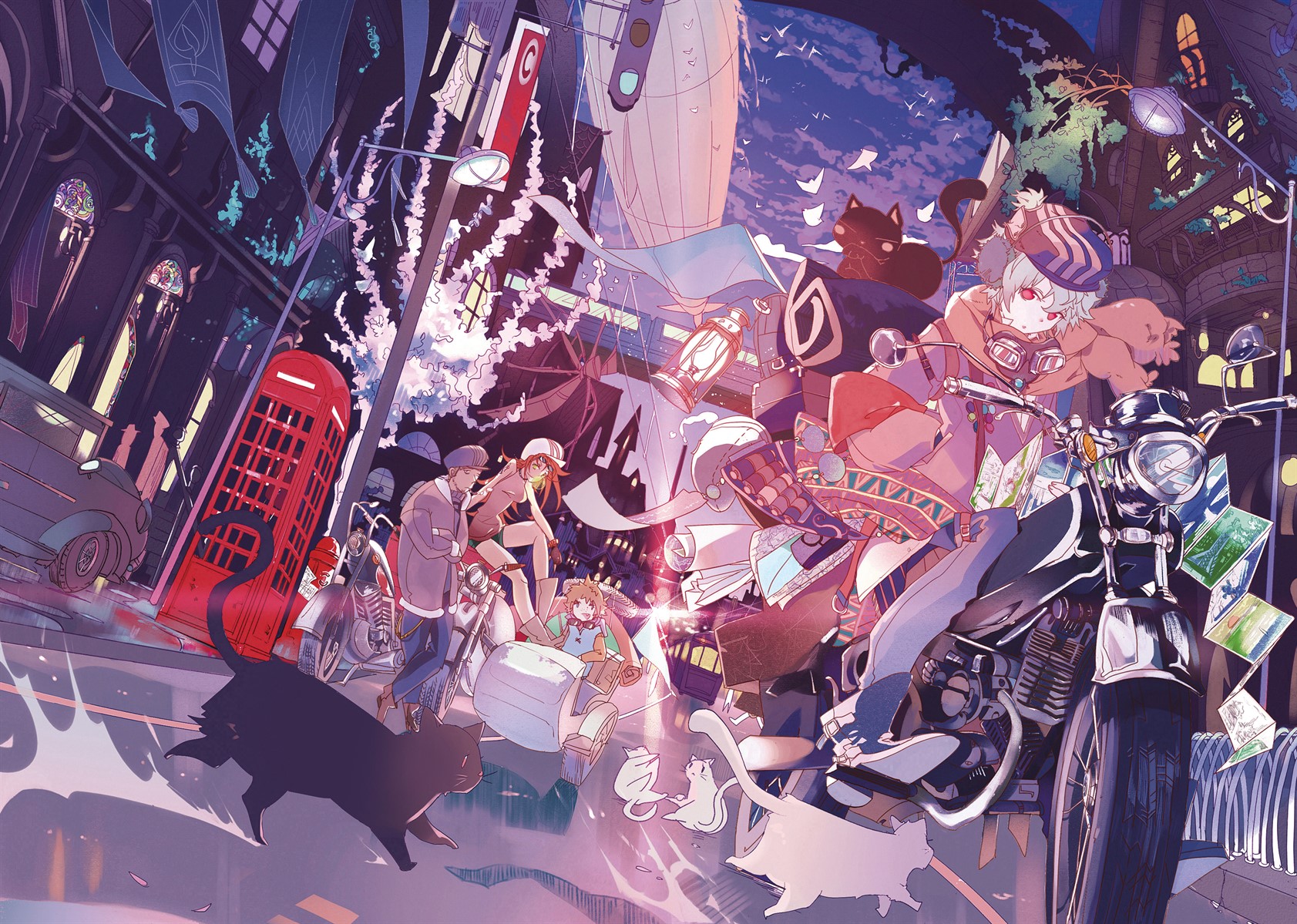
2023年5月第一次周报
C#语法
糖
模式匹配+is/not/and/or关键字
1 | int[] numbers = { 1, 2, 3 }; |
switch高级用法
1 | //Switch + Pattern matching |
record类
从 C# 9 开始,可以使用 record
关键字定义一个record
,用来提供用于封装数据的内置功能。 C# 10 允许 record class
语法作为同义词来阐明引用类型,并允许 record struct
使用相同功能定义值类型。 通过使用位置参数或标准属性语法,可以创建具有不可变属性的记录类型。
两种声明方式:
1 | public record Person(string FirstName, string LastName); |
1 | public record Person |
由于record
的值不可变,使用with
关键字,可以实现修改record
属性的效果
1 | Person person1 = new() { FirstName = "Zack", LastName = "Mount" }; |
required关键字
语言集成查询LINQ
例如,用LINQ数聊天记录里有多少个‘您’字
1 | var messages = File.ReadAllLines("D:\\聊天记录.txt"); |
或者,筛选结果
1 | int[] numbers = { 6, 68, 71, 36, 90, 12, 62, 38 }; |
甚至,使用orderby
来对筛选结果排序, ascending
是升序,descending
是降序
1 | int[] numbers = { 6, 68, 71, 36, 90, 12, 62, 38 }; |
甚至嵌套,以第一个筛选“您”为例子,可以写成更容易读的方式
1 | var messages = File.ReadAllLines("D:\\聊天记录.txt"); |
通过LINQ,查询可以写得非常骚气~代码在保持高可读性的情况下,仍然保持了优秀的效率,性能差距和传统写法并不大。
SMC
SMC,即自修改代码(Self-Modifying Code),很大程度上加大了静态分析的难度。
动态分析:因为 SMC 在运行时会改变自己的代码,因此,相比静态分析,动态分析会更有效。在运行时查看代码的变化,并在关键点设置断点,从而推断出程序流。
代码跟踪和日志记录:你可以使用工具来跟踪和记录所有执行的指令。然后你可以分析这些日志,找出代码修改和执行的模式。
绕过SMC:如果可能,可以尝试绕过SMC部分,直接获取或重构出原始的代码。SMC是通过一次性的解密或解压缩来改变代码,那么可以通过分析这个过程来获取原始代码。
逆向工程和代码分析工具:使用适合处理SMC的工具,如IDA Pro,Ghidra,radare2,binwalk,等等。这些工具可以帮助分析二进制文件,跟踪代码修改。
Session伪造/爆破
伪造脚本noraj/flask-session-cookie-manager: Flask Session Cookie Decoder/Encoder (github.com)
1 | python flask_session_cookie_manager3.py decode -s '{Secret_Key}' -c '{Session}' |
爆破脚本
1 | pip install flask-unsign # 不带字典 |
Usage
1 | flask-unsign --unsign --cookie < cookie.txt |